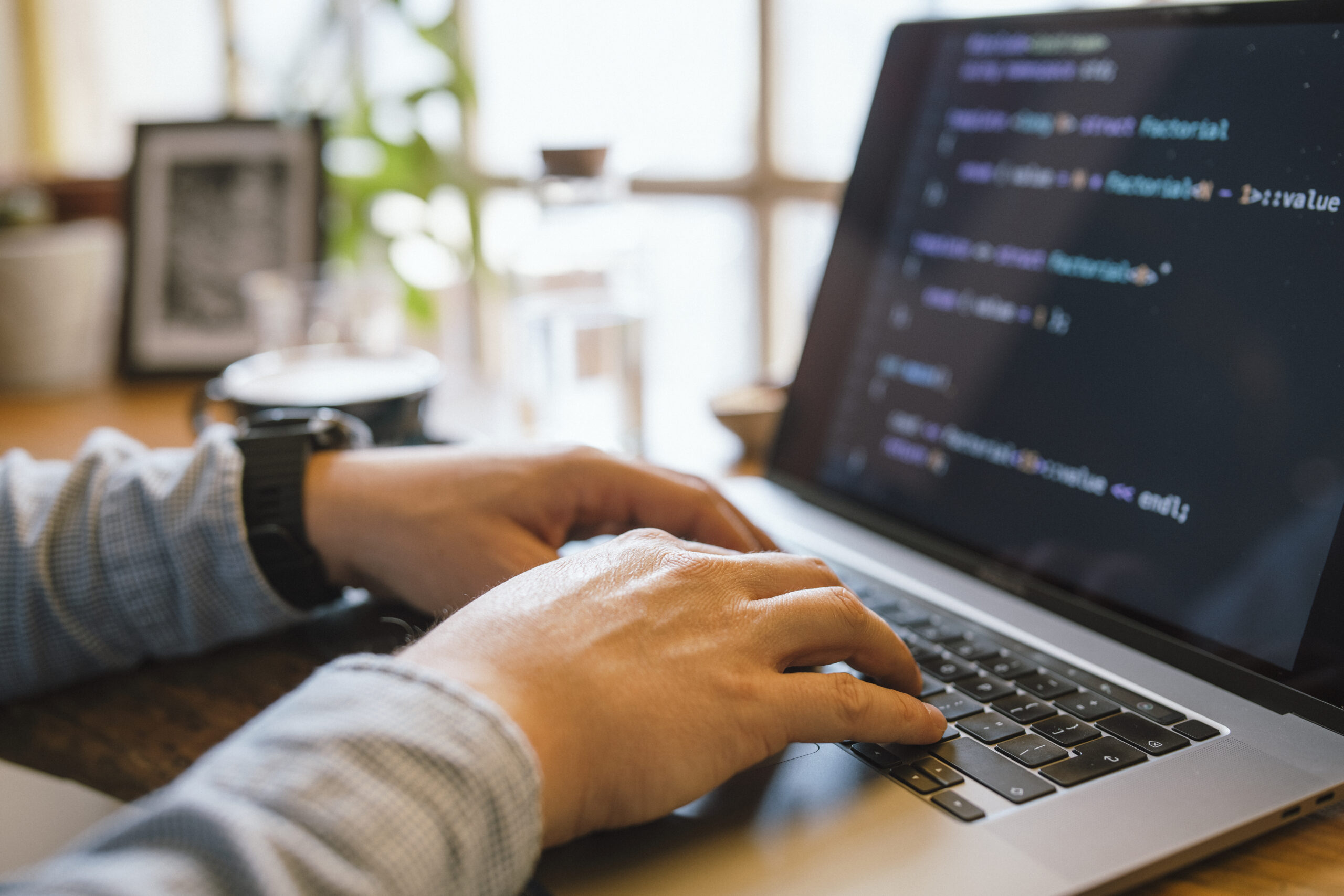
Debugging is Among the most important — still generally missed — abilities within a developer’s toolkit. It is not almost repairing damaged code; it’s about understanding how and why issues go Improper, and Understanding to Consider methodically to resolve complications competently. Whether you're a beginner or a seasoned developer, sharpening your debugging abilities can help save hrs of stress and substantially increase your productiveness. Listed below are various tactics that can help developers degree up their debugging sport by me, Gustavo Woltmann.
Learn Your Tools
One of the fastest approaches developers can elevate their debugging abilities is by mastering the applications they use everyday. While crafting code is just one Section of advancement, realizing how you can communicate with it effectively all through execution is Similarly essential. Fashionable progress environments appear Outfitted with powerful debugging abilities — but numerous builders only scratch the surface area of what these tools can perform.
Just take, for instance, an Built-in Improvement Ecosystem (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These resources assist you to set breakpoints, inspect the worth of variables at runtime, phase via code line by line, and perhaps modify code over the fly. When employed correctly, they Enable you to notice specifically how your code behaves all through execution, that's invaluable for monitoring down elusive bugs.
Browser developer tools, for example Chrome DevTools, are indispensable for entrance-stop builders. They enable you to inspect the DOM, monitor network requests, watch genuine-time effectiveness metrics, and debug JavaScript within the browser. Mastering the console, sources, and network tabs can switch disheartening UI problems into workable tasks.
For backend or program-stage developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage above functioning processes and memory administration. Discovering these tools might have a steeper Mastering curve but pays off when debugging general performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, become comfy with Edition Management units like Git to understand code background, locate the precise instant bugs were introduced, and isolate problematic modifications.
Eventually, mastering your instruments indicates going past default settings and shortcuts — it’s about building an intimate understanding of your advancement natural environment so that when issues arise, you’re not lost in the dark. The better you know your equipment, the more time you'll be able to devote solving the actual trouble rather then fumbling by means of the process.
Reproduce the issue
One of the most critical — and often missed — ways in efficient debugging is reproducing the issue. Before leaping in the code or generating guesses, developers need to create a dependable natural environment or circumstance exactly where the bug reliably seems. Without the need of reproducibility, repairing a bug will become a recreation of opportunity, normally resulting in wasted time and fragile code changes.
The initial step in reproducing a problem is accumulating as much context as possible. Talk to issues like: What actions led to the issue? Which natural environment was it in — advancement, staging, or production? Are there any logs, screenshots, or mistake messages? The more depth you've, the simpler it results in being to isolate the exact disorders beneath which the bug takes place.
As soon as you’ve gathered more than enough details, try to recreate the challenge in your local setting. This may suggest inputting exactly the same facts, simulating comparable consumer interactions, or mimicking system states. If The problem seems intermittently, contemplate producing automated assessments that replicate the edge situations or point out transitions involved. These exams not simply support expose the problem but in addition protect against regressions in the future.
At times, The problem may very well be natural environment-specific — it might come about only on sure operating methods, browsers, or beneath unique configurations. Making use of instruments like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these bugs.
Reproducing the condition isn’t only a phase — it’s a way of thinking. It necessitates patience, observation, along with a methodical strategy. But as soon as you can continually recreate the bug, you're currently halfway to fixing it. Using a reproducible situation, You need to use your debugging instruments additional effectively, test possible fixes safely, and communicate more clearly with your group or consumers. It turns an abstract complaint right into a concrete challenge — Which’s where by builders prosper.
Examine and Fully grasp the Mistake Messages
Error messages are frequently the most precious clues a developer has when some thing goes Incorrect. As an alternative to viewing them as irritating interruptions, developers should really study to deal with error messages as immediate communications in the procedure. They generally inform you what exactly happened, where it took place, and often even why it took place — if you know how to interpret them.
Get started by looking at the concept cautiously As well as in entire. Numerous developers, specially when beneath time pressure, look at the initial line and immediately start out producing assumptions. But deeper during the error stack or logs may lie the real root trigger. Don’t just duplicate and paste error messages into search engines — read and recognize them initial.
Crack the error down into areas. Is it a syntax error, a runtime exception, or a logic mistake? Does it position to a specific file and line variety? What module or perform activated it? These questions can information your investigation and level you towards the responsible code.
It’s also valuable to understand the terminology on the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically follow predictable styles, and learning to recognize these can considerably speed up your debugging approach.
Some faults are vague or generic, and in those circumstances, it’s important to look at the context by which the error transpired. Look at associated log entries, input values, and up to date changes inside the codebase.
Don’t forget compiler or linter warnings possibly. These frequently precede more substantial difficulties and supply hints about likely bugs.
In the long run, mistake messages are not your enemies—they’re your guides. Studying to interpret them appropriately turns chaos into clarity, supporting you pinpoint difficulties faster, decrease debugging time, and turn into a additional economical and self-assured developer.
Use Logging Wisely
Logging is Probably the most effective equipment within a developer’s debugging toolkit. When utilised correctly, it offers serious-time insights into how an software behaves, encouraging you have an understanding of what’s going on underneath the hood without having to pause execution or step through the code line by line.
A good logging system starts off with knowing what to log and at what amount. Popular logging degrees include things like DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for in depth diagnostic info throughout development, Facts for normal events (like thriving start out-ups), WARN for prospective issues that don’t crack the appliance, ERROR for precise problems, and Lethal if the program can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant info. Too much logging can obscure vital messages and decelerate your technique. Concentrate on key situations, condition modifications, enter/output values, and demanding conclusion factors as part of your code.
Format your log messages Evidently and constantly. Include context, for instance timestamps, request IDs, and performance names, so it’s easier to trace difficulties in dispersed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs let you observe how variables evolve, what circumstances are fulfilled, and what branches of logic are executed—all with out halting This system. They’re Specifically important in creation environments where by stepping by means of code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with monitoring dashboards.
In the end, clever logging is about equilibrium and clarity. Having a perfectly-believed-out logging tactic, you can decrease the time it will require to identify troubles, attain deeper visibility into your programs, and Enhance the In general maintainability and reliability of the code.
Assume Similar to a Detective
Debugging is not only a complex endeavor—it's a type of investigation. To properly detect and fix bugs, developers need to technique the process like a detective solving a mystery. This frame of mind will help stop working elaborate issues into manageable components and comply with clues logically to uncover the foundation induce.
Get started by gathering evidence. Look at the signs and symptoms of the trouble: error messages, incorrect output, or functionality challenges. Identical to a detective surveys against the law scene, obtain just as much applicable information and facts as you could without leaping to conclusions. Use logs, exam conditions, and user experiences to piece alongside one another a transparent photo of what’s taking place.
Up coming, type hypotheses. Inquire your self: What might be leading to this conduct? Have any modifications recently been built into the codebase? Has this challenge transpired just before under identical circumstances? The intention will be to slim down choices and determine likely culprits.
Then, test your theories systematically. Try to recreate the condition in a very controlled natural environment. When you suspect a particular function or ingredient, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, question your code concerns and Enable the results guide you closer to the reality.
Shell out close interest to small aspects. Bugs typically hide from the the very least anticipated sites—just like a lacking semicolon, an off-by-one particular error, or possibly a race situation. Be extensive and affected person, resisting the urge to patch The difficulty with no fully knowledge it. Short term fixes may perhaps conceal the actual issue, just for it to resurface afterwards.
And finally, keep notes on Anything you attempted and figured out. Just as detectives log their investigations, documenting your debugging approach can help you save time for potential difficulties and assist Other folks have an understanding of your reasoning.
By pondering similar to a detective, developers can sharpen their analytical capabilities, solution issues methodically, and turn into more practical at uncovering concealed problems in intricate units.
Write Assessments
Crafting tests is one of the best solutions to help your debugging abilities and All round growth performance. Checks don't just aid capture bugs early and also function a security net that gives you self-assurance when generating modifications to your codebase. A nicely-analyzed software is much easier to debug mainly because it allows you to pinpoint exactly exactly where and when an issue occurs.
Start with unit checks, which focus on personal functions or modules. These little, isolated tests can rapidly reveal whether or not a specific bit of logic is Doing the job as envisioned. Every time a take a look at fails, you quickly know wherever to glance, appreciably minimizing time invested debugging. Unit checks are In particular valuable for catching regression bugs—troubles that reappear right after previously getting fixed.
Future, combine integration assessments and stop-to-finish checks into your workflow. These enable be certain that numerous parts of your software perform collectively easily. They’re significantly handy for catching bugs that take place in complex units with several components or expert services interacting. If one thing breaks, your tests can inform you which Section of the pipeline failed and underneath what circumstances.
Crafting exams also forces you to definitely Feel critically regarding your code. To test a aspect appropriately, you need to be aware of its inputs, expected outputs, and edge conditions. This amount of understanding In a natural way leads to higher code composition and fewer bugs.
When debugging a concern, crafting a failing check that reproduces the bug is usually a powerful initial step. Once the examination fails continuously, it is possible to deal with fixing the bug and look at your exam pass when The problem is solved. This approach ensures that the exact same bug doesn’t return Down the road.
Briefly, crafting tests turns debugging from a annoying guessing activity into a structured and predictable procedure—supporting you capture extra bugs, quicker and even more reliably.
Acquire Breaks
When debugging a tough problem, it’s straightforward to be immersed in the situation—gazing your screen for hours, attempting Remedy soon after Option. But One of the more underrated debugging applications is simply stepping absent. Using breaks aids you reset your brain, lessen stress, and sometimes see The problem from a new viewpoint.
When you are also near to the code for also extended, cognitive fatigue sets in. You could start out overlooking evident faults or misreading code that you choose to wrote just several hours previously. In this particular condition, your brain gets to be less efficient at trouble-resolving. A brief walk, a coffee break, or even switching to a different endeavor for ten–15 minutes can refresh your concentrate. Many builders report acquiring the basis of a difficulty after they've taken time to disconnect, permitting their subconscious do the job from the track record.
Breaks also assist prevent burnout, Primarily through for a longer time debugging sessions. Sitting down in front of a monitor, mentally caught, is not just unproductive but will also draining. Stepping absent enables you to return with renewed Power in addition to a clearer frame of mind. You could suddenly detect a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you ahead of.
If you’re caught, a great general guideline would be to established a timer—debug actively for 45–sixty minutes, then take a five–10 moment break. Use that point to move all around, stretch, or do anything unrelated to code. It may come to feel counterintuitive, especially beneath limited Developers blog deadlines, nevertheless it basically brings about quicker and simpler debugging in the long run.
In a nutshell, having breaks isn't an indication of weak spot—it’s a smart approach. It presents your brain Room to breathe, increases your perspective, and will help you steer clear of the tunnel vision That always blocks your development. Debugging is really a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Each bug you come across is much more than simply a temporary setback—It is a chance to improve to be a developer. No matter if it’s a syntax mistake, a logic flaw, or a deep architectural problem, each can train you a thing important in the event you take some time to mirror and assess what went Completely wrong.
Start by asking yourself a couple of crucial inquiries when the bug is fixed: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with much better methods like unit screening, code evaluations, or logging? The solutions usually reveal blind spots in your workflow or comprehending and assist you to Develop stronger coding behavior relocating forward.
Documenting bugs may also be a great habit. Keep a developer journal or maintain a log in which you Observe down bugs you’ve encountered, the way you solved them, and Whatever you realized. As time passes, you’ll start to see styles—recurring troubles or widespread blunders—that you could proactively keep away from.
In group environments, sharing what you've learned from the bug using your peers is usually In particular strong. Regardless of whether it’s through a Slack information, a short write-up, or A fast information-sharing session, helping Some others stay away from the same issue boosts workforce effectiveness and cultivates a stronger Mastering culture.
Extra importantly, viewing bugs as lessons shifts your mindset from annoyance to curiosity. As opposed to dreading bugs, you’ll get started appreciating them as vital parts of your progress journey. In the end, a lot of the greatest builders usually are not those who create perfect code, but individuals who constantly study from their errors.
In the long run, Every bug you deal with adds a fresh layer towards your skill set. So future time you squash a bug, take a minute to replicate—you’ll come away a smarter, additional capable developer as a result of it.
Summary
Bettering your debugging competencies requires time, follow, and tolerance — however the payoff is big. It would make you a far more efficient, assured, and able developer. Another time you're knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be better at Everything you do.